题目:
创建一颗二叉树,并进行广度优先遍历
输入格式:
abd##e##cf###,#代表空节点
输出格式:
按广度优先顺序输出,两个字符间有一个空格
输入样例:
在这里给出一组输入。例如:
输出样例:
在这里给出相应的输出。例如:
注意:最后一个没有空格
1.构建结构体
1 2 3 4 5 6 7 8 9
| struct node { char data; node* left; node* right; }; typedef node* Node;
|
2.全局变量
1 2 3
| string s; queue<node*> q; int i=0;
|
3.创建二叉树
1 2 3 4 5 6 7 8 9 10 11 12 13
| void init(Node* T){ if(s[i]=='#'){ i++; *T=NULL; } else{ (*T)=new node; (*T)->data=s[i]; i++; init(&(*T)->left); init(&(*T)->right); } }
|
3.用队列进行层序遍历
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| void show(Node node){ Node t; if(node!=NULL){ q.push(node); int flag=1; while(!q.empty()){ t=q.front(); q.pop(); if(flag){ flag=0; cout<<t->data; }else{ cout<<" "<<t->data; } if(t->lchild!=NULL) q.push(t->left); if(t->rchild!=NULL) q.push(t->right); delete t; } } }
|
4.完整程序
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| #include<iostream> #include<string> #include<queue> using namespace std; struct node { char data; node* left; node* right; }; typedef node* Node; string s; queue<node*> q; int i=0; void init(Node* T){ if(s[i]=='#'){ i++; *T=NULL; } else{ (*T)=new node; (*T)->data=s[i]; i++; init(&(*T)->left); init(&(*T)->right); } } void show(Node node){ Node t; if(node!=NULL){ q.push(node); int flag=1; while(!q.empty()){ t=q.front(); q.pop(); if(flag){ flag=0; cout<<t->data; }else{ cout<<" "<<t->data; } if(t->left!=NULL) q.push(t->left); if(t->right!=NULL) q.push(t->right); delete t; } } } int main(){ getline(cin,s); Node* root=new Node; Node rr=NULL; init(&rr); show(rr); delete root; return 0; }
|
pta测试:
5ms 480kb
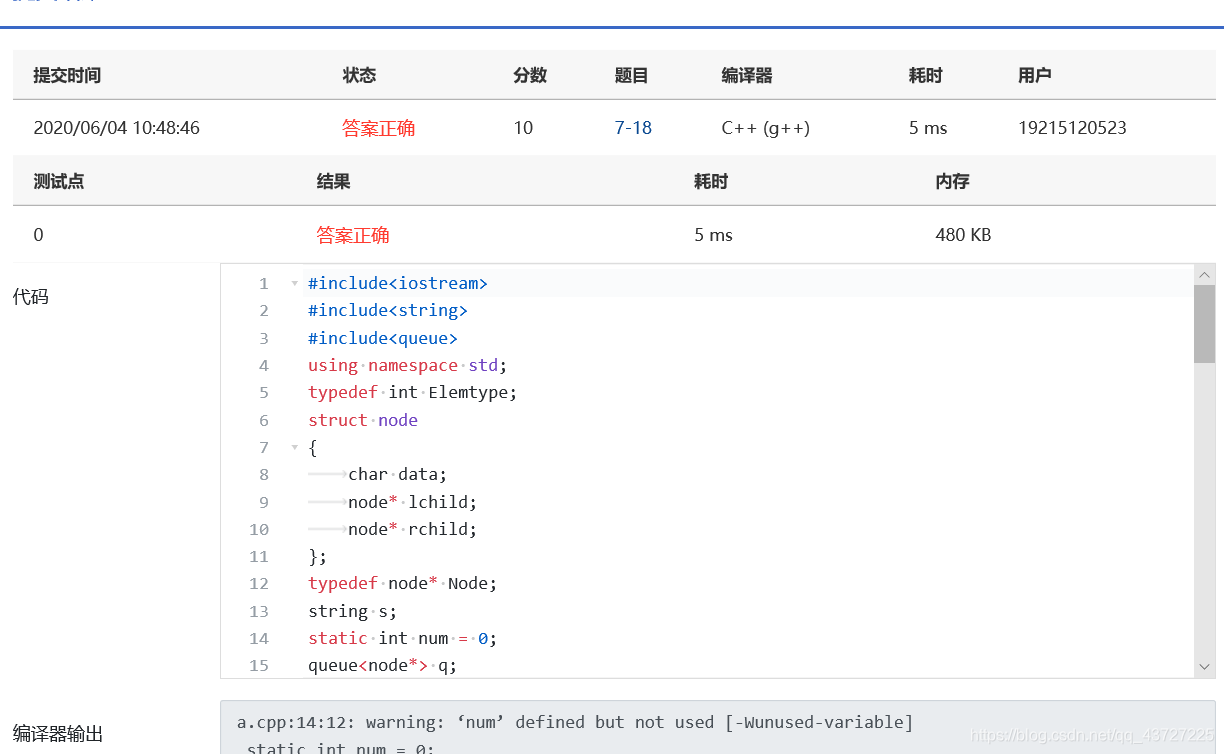